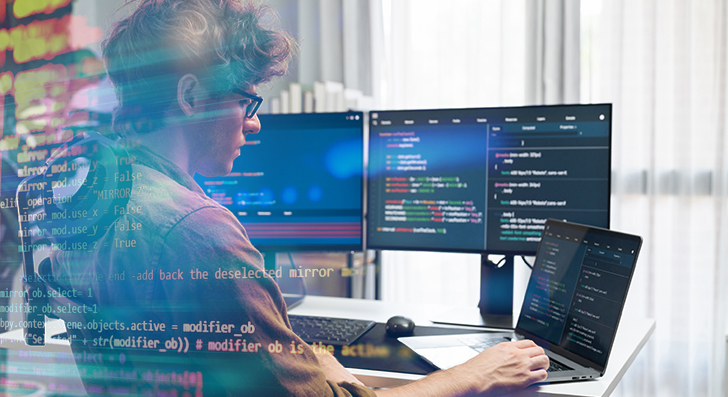
Scalability usually means your application can deal with advancement—additional end users, much more details, plus much more targeted traffic—without having breaking. As a developer, making with scalability in mind will save time and pressure later. Below’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later—it ought to be portion of your prepare from the start. Several purposes fall short every time they expand speedy mainly because the original layout can’t handle the extra load. To be a developer, you should Assume early about how your technique will behave under pressure.
Start off by designing your architecture for being adaptable. Avoid monolithic codebases the place all the things is tightly connected. Alternatively, use modular structure or microservices. These designs crack your application into smaller, impartial sections. Each module or services can scale on its own devoid of influencing The entire process.
Also, think about your database from day one particular. Will it will need to take care of a million customers or maybe 100? Choose the correct sort—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t require them but.
A further important stage is to prevent hardcoding assumptions. Don’t compose code that only operates beneath recent problems. Contemplate what would transpire In case your user foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use layout designs that help scaling, like message queues or event-driven techniques. These assist your app handle more requests without having overloaded.
After you Make with scalability in your mind, you are not just getting ready for success—you're lessening long run complications. A effectively-planned system is less complicated to keep up, adapt, and increase. It’s far better to prepare early than to rebuild afterwards.
Use the appropriate Database
Choosing the ideal databases is actually a key Element of setting up scalable apps. Not all databases are developed the same, and utilizing the Improper you can sluggish you down or perhaps induce failures as your app grows.
Commence by comprehending your details. Could it be highly structured, like rows inside a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient fit. These are definitely potent with associations, transactions, and regularity. Additionally they aid scaling tactics like read replicas, indexing, and partitioning to manage more website traffic and information.
If the knowledge is more versatile—like person activity logs, merchandise catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured details and may scale horizontally additional easily.
Also, take into account your browse and create designs. Are you presently performing a great deal of reads with much less writes? Use caching and read replicas. Will you be managing a hefty publish load? Take a look at databases that can manage significant generate throughput, or perhaps function-dependent data storage techniques like Apache Kafka (for momentary facts streams).
It’s also good to Believe ahead. You may not want State-of-the-art scaling options now, but deciding on a databases that supports them implies you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your details depending on your access styles. And always check database efficiency while you expand.
In brief, the correct database depends upon your app’s structure, velocity requires, and how you anticipate it to grow. Take time to select correctly—it’ll help save a great deal of problems later.
Optimize Code and Queries
Speedy code is essential to scalability. As your app grows, just about every modest delay adds up. Improperly published code or unoptimized queries can slow down overall performance and overload your method. That’s why it’s important to Establish successful logic from the start.
Commence by creating clean up, uncomplicated code. Keep away from repeating logic and remove anything unwanted. Don’t select the most complex Option if an easy one is effective. Keep the functions short, centered, and easy to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes way too long to operate or employs an excessive amount of memory.
Upcoming, examine your databases queries. These usually gradual issues down much more than the code itself. Ensure that Each and every question only asks for the data you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative pick out particular fields. Use indexes to hurry up lookups. And avoid carrying out a lot of joins, Particularly across large tables.
In case you see exactly the same knowledge being requested over and over, use caching. Retail store the outcomes briefly working with tools like Redis or Memcached which means you don’t should repeat costly operations.
Also, batch your databases functions after you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to check with huge datasets. Code and queries that work good with 100 information may possibly crash whenever they have to manage one million.
To put it briefly, scalable applications are speedy applications. Keep the code limited, your queries lean, and use caching when needed. These steps support your software keep clean and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more consumers and a lot more targeted traffic. If almost everything goes by way of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two resources assist keep your application rapid, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of 1 server doing all the do the job, the load balancer routes people to diverse servers depending on availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-centered solutions from AWS and Google Cloud make this straightforward to create.
Caching is about storing information quickly so it could be reused rapidly. When buyers request the same information and facts once again—like a product site or even a profile—you don’t need to fetch it with the database every time. You may serve it within the cache.
There are 2 popular forms of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for fast entry.
two. Consumer-facet caching (like browser caching or CDN caching) outlets static information near the user.
Caching lowers databases load, enhances velocity, and tends to make your application more productive.
Use caching for things which don’t alter generally. And always be sure your cache is current when facts does change.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they help your application tackle much more end users, continue to be quick, and Recuperate from challenges. If you plan to develop, you need the two.
Use Cloud and Container Instruments
To make scalable applications, you will need instruments that permit your application grow very easily. That’s the place cloud platforms and containers can be found in. They offer you adaptability, decrease setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t should obtain components or guess upcoming potential. When targeted visitors increases, you can add much more resources with just a few clicks or immediately employing automobile-scaling. When here targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to focus on building your application in place of taking care of infrastructure.
Containers are One more crucial Instrument. A container packages your application and all the things it ought to operate—code, libraries, settings—into one device. This causes it to be straightforward to move your application among environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of a number of containers, instruments like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If a single part within your app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your application into companies. You are able to update or scale pieces independently, which can be perfect for functionality and reliability.
Briefly, making use of cloud and container tools signifies you can scale rapidly, deploy effortlessly, and Get well quickly when troubles happen. If you need your application to expand without the need of limitations, start out utilizing these equipment early. They help you save time, minimize possibility, and assist you to continue to be focused on creating, not correcting.
Observe Everything
If you don’t watch your application, you won’t know when factors go wrong. Monitoring aids the thing is how your application is performing, place troubles early, and make improved decisions as your app grows. It’s a essential Section of setting up scalable methods.
Commence by monitoring primary metrics like CPU use, memory, disk space, and response time. These tell you how your servers and providers are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—keep an eye on your application way too. Control how much time it's going to take for customers to load pages, how frequently faults materialize, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. For example, if your reaction time goes higher than a Restrict or maybe a assistance goes down, you must get notified quickly. This will help you correct troubles quickly, frequently ahead of consumers even recognize.
Monitoring is usually handy if you make adjustments. In the event you deploy a new aspect and find out a spike in problems or slowdowns, you are able to roll it again in advance of it brings about genuine destruction.
As your application grows, site visitors and data raise. Without having monitoring, you’ll miss out on signs of hassle right up until it’s far too late. But with the correct tools in position, you stay on top of things.
In brief, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about knowing your procedure and ensuring it really works effectively, even stressed.
Last Views
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By coming up with meticulously, optimizing sensibly, and using the suitable resources, you may Develop applications that mature easily devoid of breaking stressed. Commence smaller, think huge, and Make smart.